完全二叉树的节点个数
题目描述:
求一个完全二叉树的节点个数。 leetcode链接
解题
1.通用解法
对于普通的二叉树,我们可以利用深度优先和广度优先方式,在遍历节点的过程中统计节点的个数,在此题中也可以采用这种方式,下面给出代码,不过多赘述。
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
public int countNodes(TreeNode root) { if(root== null) return 0; return countNodes(root.left)+countNodes(root.right)+1; }
public int countNodes(TreeNode root) { if (root == null) return 0; Queue<TreeNode> queue = new LinkedList<>(); queue.add(root); int res = 0; while (!queue.isEmpty()) { int size = queue.size(); for(int i=0;i<size;i++){ TreeNode node = queue.poll(); res++; if (node.left != null) queue.offer(cur.left); if (node.right != null) queue.offer(cur.right); } } return res; }
|
2.完全二叉树解法
但是这并没有达到本题考察的目的
,题目中指出该二叉树为完全二叉树
[^1],所以应该利用其特点来解题。我们知道满二叉树的计算公式是:2的深度次方-1
,而一棵完全二叉树虽然无法直接利用该公式计算,但在它的左子树或右子树中一定可以找到满二叉树,而那部分完全可以借助满二叉树的计算公式。具体思路以注释形式体现在代码中。
方框中为在子树中找到的满二叉树:
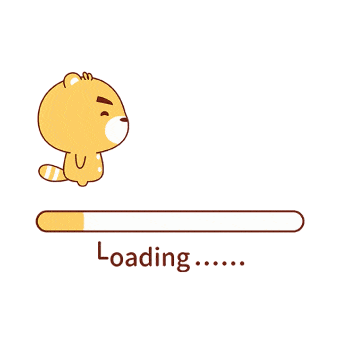
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
public int countNodes(TreeNode root) { if(root== null) return 0; int leftDepth=1; int rightDepth=1; TreeNode left=root.left; TreeNode right=root.right; while(left!=null){ left=left.left; leftDepth++; } while(right!=null){ right=right.right; rightDepth++; } if(leftDepth==rightDepth){ return (int)(Math.pow(2,leftDepth) -1); } return countNodes(root.left)+countNodes(root.right)+1; }
|
[^1]: 设二叉树的深度为h
,除第 h 层外
,其它各层 (1~h-1) 的结点数都达到最大个数(即1~h-1层为一个满二叉 树)
,第 h 层所有的结点都连续集中在最左边,这就是完全二叉树